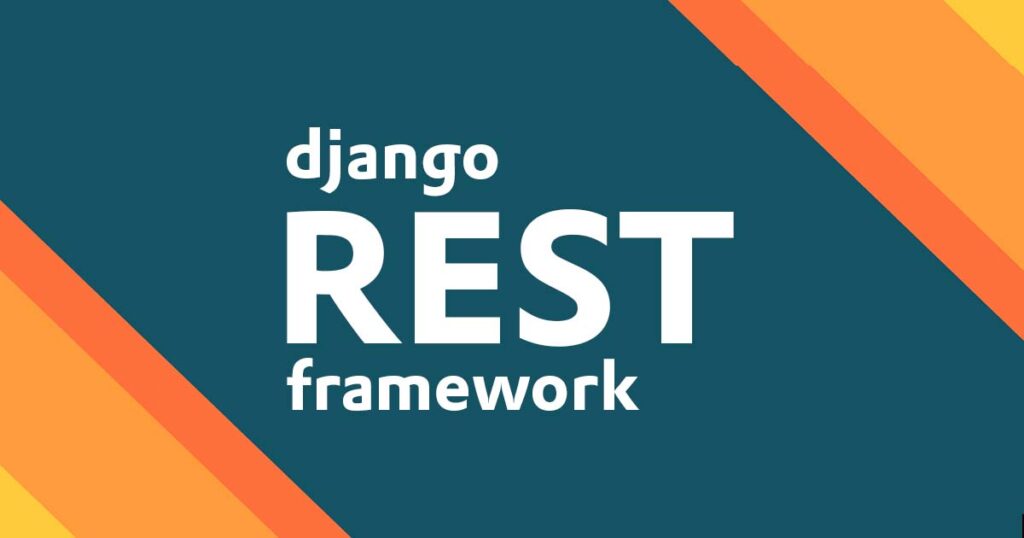
Django Channels is a powerful, open-source tool that allows for real-time communication between web applications and servers using websockets. Channels extends Django to handle the asynchronous protocols of websockets, providing a framework for building interactive applications such as chat rooms, multiplayer games, and real-time analytics.
In this blog post, we’ll take a closer look at Django Channels and explore how it can be used to build real-time web applications.
What are Websockets?
Before we dive into DjangoChannels, let’s first understand what websockets are. Websockets are a protocol that allows for two-way communication between a web browser and a server in real-time. This is different from traditional HTTP requests, which are one-way and require a new connection to be established for each request.
Websockets use a persistent connection between the browser and server, allowing for real-time updates to be sent and received without the need for constant refreshing or polling. This makes them ideal for applications that require real-time updates, such as chat applications or multiplayer games.
What is Django Channels?
DjangoChannels is a third-party package that allows for the integration of websockets into Django applications. It provides a framework for building real-time applications using websockets, as well as support for other asynchronous protocols such as HTTP long-polling and Server-Sent Events (SSE).
DjangoChannels includes an interface for working with websockets, as well as a system for routing incoming messages to the appropriate consumers (similar to views in Django). It also provides support for message queuing, allowing for reliable message delivery even under heavy load.
How to Use Django Channels
To use DjangoChannels in your application, you’ll first need to install it using pip:
pip install channels
Once installed, you can add Channels to your Django project by including it in your INSTALLED_APPS
setting:
# settings.py
INSTALLED_APPS = [
# ...
'channels',
]
Next, you’ll need to configure Channels to use the appropriate backend for your application. Channels supports several backends, including Redis and in-memory channels. For this example, we’ll use Redis:
# settings.py
CHANNEL_LAYERS = {
"default": {
"BACKEND": "channels_redis.core.RedisChannelLayer",
"CONFIG": {
"hosts": [("localhost", 6379)],
},
},
}
With Channels installed and configured, you can now define your consumers. Consumers are similar to views in Django and handle incoming websocket messages. Here’s an example consumer that echoes any incoming messages back to the sender:
# consumers.py
from channels.generic.websocket import WebsocketConsumer
import json
class EchoConsumer(WebsocketConsumer):
def connect(self):
self.accept()
def disconnect(self, close_code):
pass
def receive(self, text_data):
data = json.loads(text_data)
self.send(text_data=json.dumps({
'message': data['message']
}))
In this example, the connect()
method is called when a client connects to the websocket, disconnect()
is called when the client disconnects, and receive()
is called when a message is received.
Finally, you’ll need to define your routing for incoming messages. This tells Channels which consumer should handle each incoming message. Here’s an example routing configuration that routes all messages to the EchoConsumer
:
# routing.py
from django.urls import re_path
from . import consumers
websocket_urlpatterns = [
re_path(r'ws/echo/$', consumers.EchoConsumer.as_asgi()),
]
GitHub Link: