Let’s explore an example of Python Django Ajax CRUD Operations. Ajax is a method that adds dynamism to web development. It enables loading data from servers without needing to refresh web pages. AJAX is short for Asynchronous JavaScript and XML.
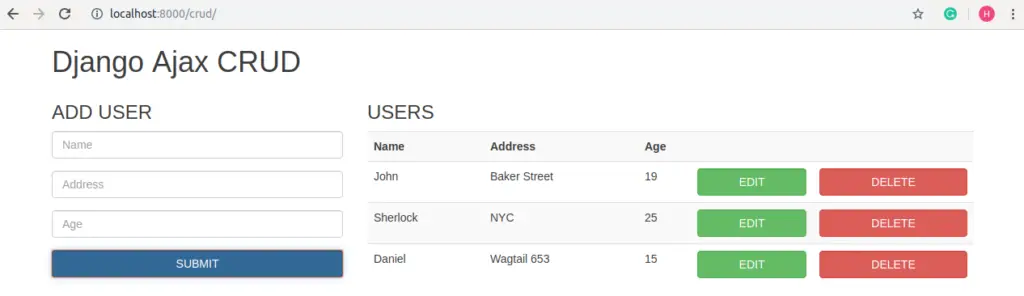
In this tutorial, we’ll discover how to perform CRUD operations using Django, Ajax Form Submission, and JSON. We’ll utilize Class-Based Views Functions for this purpose. CRUD involves the fundamental actions we perform on databases: Create, Read, Update, and Delete. We’ll illustrate this through a User Management System. Here, we’ll learn how to add, update, and delete user information. You can find the complete source code on Github, link at end of the blog.
Read our New Blog on Task Management App using Django AJAX CRUD.
Step by Step Implementation
Step 1: Django Project Setup
Before we begin, ensure you have the following prerequisites in place:
- Django installed on your system.
- JQuery Understanding
- Basic familiarity with Django project structure.
Note: For this tutorial, we are using our basic skeleton project for Django. You can also download the project from here.
We have created the project with name “myproject” and app name “myapp‘”. Inside “myapp” we have created a folder named “templates” for storing our html templates.
Step 2: Basic Configurations
Lets start by creating base.html
which we will be inheriting in other html files in templates
folder. We are going to use JQuery for implementing Ajax requests and Bootstrap for a beautiful user interface
<!-- myapp/templates/base.html -->
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>{% block title %}Title{% endblock title %}</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous">
{% block stylesheet %}{% endblock stylesheet %}
</head>
<body>
<main>
{% block content %}
{% endblock content %}
</main>
<script src='https://code.jquery.com/jquery-3.2.1.min.js'></script>
<script src='https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js'></script>
{% block javascript %}
{% endblock javascript%}
</body>
</html>
Now lets create model for storing data. In your app, you will find models.py
. Add the following code in it.
# myapp/models.py
from django.db import models
class CrudUser(models.Model):
name = models.CharField(max_length=30, blank=True)
address = models.CharField(max_length=100, blank=True)
age = models.IntegerField(blank=True, null=True)
Step 3: Create View and URL’s for Listing, Create, Read, Update and Delete Objects
We will creating CrudView
for loading all user details. CreateCrudUser
, DeleteCrudUser
, UpdateCrudUser
views for creating, updating and listing users which we will be using to interact with AJAX system.
# myapp/views.py
from django.shortcuts import render
from django.urls import reverse_lazy
from .models import CrudUser
from django.views.generic import TemplateView, View, DeleteView
from django.core import serializers
from django.http import JsonResponse
class CrudView(TemplateView):
template_name = 'crud.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['users'] = CrudUser.objects.all()
return context
class CreateCrudUser(View):
def get(self, request):
name1 = request.GET.get('name', None)
address1 = request.GET.get('address', None)
age1 = request.GET.get('age', None)
obj = CrudUser.objects.create(
name = name1,
address = address1,
age = age1
)
user = {'id':obj.id,'name':obj.name,'address':obj.address,'age':obj.age}
data = {
'user': user
}
return JsonResponse(data)
class DeleteCrudUser(View):
def get(self, request):
id1 = request.GET.get('id', None)
CrudUser.objects.get(id=id1).delete()
data = {
'deleted': True
}
return JsonResponse(data)
class UpdateCrudUser(View):
def get(self, request):
id1 = request.GET.get('id', None)
name1 = request.GET.get('name', None)
address1 = request.GET.get('address', None)
age1 = request.GET.get('age', None)
obj = CrudUser.objects.get(id=id1)
obj.name = name1
obj.address = address1
obj.age = age1
obj.save()
user = {'id':obj.id,'name':obj.name,'address':obj.address,'age':obj.age}
data = {
'user': user
}
return JsonResponse(data)
Now create a urls.py
file in your app, to to comunicate with views.
# myapp/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('crud/', views.CrudView.as_view(), name='crud_ajax'),
path('ajax/crud/create/', views.CreateCrudUser.as_view(), name='crud_ajax_create'),
path('ajax/crud/delete/', views.DeleteCrudUser.as_view(), name='crud_ajax_delete'),
path('ajax/crud/update/', views.UpdateCrudUser.as_view(), name='crud_ajax_update'),
]
And in your main projects urls.py include it.
# myproject/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('myapp.urls')),
]
Step 4: Create HTML UI for Ajax
In templates folder, create a file named “crud.html“.
{% extends 'base.html' %}
{% load static %}
{% block title %}Django Ajax CRUD{% endblock %}
{% block content %}
<div class="container">
<h1>Django Ajax CRUD</h1>
<div class="row">
<div class="col-md-4 ">
<h3>ADD USER</h3>
<form id="addUser" action="">
<div class="form-group">
<input class="form-control" type="text" name="name" placeholder="Name" required>
</div>
<div class="form-group">
<input class="form-control" type="text" name="address" placeholder="Address" required>
</div>
<div class="form-group">
<input class="form-control" type="number" name="age" min="10" max="100" placeholder="Age" required>
</div>
<button class="btn btn-primary form-control" type="submit">SUBMIT</button>
</form>
</div>
<div class="col-md-8">
<h3>USERS</h3>
<table id="userTable" class="table table-striped">
<tr>
<th>Name</th>
<th>Address</th>
<th colspan="3">Age</th>
</tr>
{% for user in users %}
<tr id="user-{{user.id}}">
<td class="userName userData" name="name">{{user.name}}</td>
<td class="userAddress userData" name="address">{{user.address}}</td>
<td class="userAge userData" name="age">{{user.age}}</td>
<td align="center">
<button class="btn btn-success form-control" onClick="editUser({{user.id}})" data-toggle="modal" data-target="#myModal")">EDIT</button>
</td>
<td align="center">
<button class="btn btn-danger form-control" onClick="deleteUser({{user.id}})">DELETE</button>
</td>
</tr>
{% endfor %}
</table>
</div>
</div>
</div>
<!-- Modal -->
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<h4 class="modal-title" id="myModalLabel">Update User</h4>
</div>
<form id="updateUser" action="">
<div class="modal-body">
<input class="form-control" id="form-id" type="hidden" name="formId"/>
<label for="name">Name</label>
<input class="form-control" id="form-name" type="text" name="formName"/>
<label for="address">Address</label>
<input class="form-control" id="form-address" type="text" name="formAddress"/>
<label for="age">Age</label>
<input class="form-control" id="form-age" type="number" name="formAge" min=10 max=100/>
</div>
<div class="modal-footer">
<button type="submit" class="btn btn-primary" >Save changes</button>
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</form>
</div>
</div>
</div>
{% endblock %}
{% block javascript %}
<script>
// Create Django Ajax Call
$("form#addUser").submit(function() {
var nameInput = $('input[name="name"]').val().trim();
var addressInput = $('input[name="address"]').val().trim();
var ageInput = $('input[name="age"]').val().trim();
if (nameInput && addressInput && ageInput) {
// Create Ajax Call
$.ajax({
url: '{% url "crud_ajax_create" %}',
data: {
'name': nameInput,
'address': addressInput,
'age': ageInput
},
dataType: 'json',
success: function (data) {
if (data.user) {
appendToUsrTable(data.user);
}
}
});
} else {
alert("All fields must have a valid value.");
}
$('form#addUser').trigger("reset");
return false;
});
// Delete Django Ajax Call
function deleteUser(id) {
var action = confirm("Are you sure you want to delete this user?");
if (action != false) {
$.ajax({
url: '{% url "crud_ajax_delete" %}',
data: {
'id': id,
},
dataType: 'json',
success: function (data) {
if (data.deleted) {
$("#userTable #user-" + id).remove();
}
}
});
}
}
// Create Django Ajax Call
$("form#updateUser").submit(function() {
var idInput = $('input[name="formId"]').val().trim();
var nameInput = $('input[name="formName"]').val().trim();
var addressInput = $('input[name="formAddress"]').val().trim();
var ageInput = $('input[name="formAge"]').val().trim();
if (nameInput && addressInput && ageInput) {
// Create Ajax Call
$.ajax({
url: '{% url "crud_ajax_update" %}',
data: {
'id': idInput,
'name': nameInput,
'address': addressInput,
'age': ageInput
},
dataType: 'json',
success: function (data) {
if (data.user) {
updateToUserTabel(data.user);
}
}
});
} else {
alert("All fields must have a valid value.");
}
$('form#updateUser').trigger("reset");
$('#myModal').modal('hide');
return false;
});
// Update Django Ajax Call
function editUser(id) {
if (id) {
tr_id = "#user-" + id;
name = $(tr_id).find(".userName").text();
address = $(tr_id).find(".userAddress").text();
age = $(tr_id).find(".userAge").text();
$('#form-id').val(id);
$('#form-name').val(name);
$('#form-address').val(address);
$('#form-age').val(age);
}
}
function appendToUsrTable(user) {
$("#userTable > tbody:last-child").append(`
<tr id="user-${user.id}">
<td class="userName" name="name">${user.name}</td>
'<td class="userAddress" name="address">${user.address}</td>
'<td class="userAge" name="age">${user.age}</td>
'<td align="center">
<button class="btn btn-success form-control" onClick="editUser(${user.id})" data-toggle="modal" data-target="#myModal")">EDIT</button>
</td>
<td align="center">
<button class="btn btn-danger form-control" onClick="deleteUser(${user.id})">DELETE</button>
</td>
</tr>
`);
}
function updateToUserTabel(user){
$("#userTable #user-" + user.id).children(".userData").each(function() {
var attr = $(this).attr("name");
if (attr == "name") {
$(this).text(user.name);
} else if (attr == "address") {
$(this).text(user.address);
} else {
$(this).text(user.age);
}
});
}
</script>
{% endblock javascript %}
Step 5: Run Migrations and Test your Application
Now you need, create and make migrations. In your terminal, run the following commands.
python manage.py makemigrations
python manage.py migrate
After migrations, you need to run your Django application, using below command
python manage.py runserver
And go to http://localhost:8000/crud/
to see your application.
Conclusion
In conclusion, this tutorial has provided a clear insight into Python Django Ajax CRUD Operations. By harnessing the power of Ajax, we’ve unlocked dynamic capabilities in web development, allowing data retrieval from servers without page reloads. The tutorial focused on essential CRUD operations – Create, Read, Update, and Delete – utilizing Django’s Class-Based Views Functions. This knowledge has been applied to a User Management System, showcasing the process of adding, updating, and deleting user details. By mastering these concepts, you’re empowered to create more interactive and user-friendly web applications.
Find this tutorial on Github.