When developing a Django project, organizing your codebase and folders effectively is crucial for maintainability, scalability, and collaboration. A well-structured folder and directory layout ensures a clean separation of concerns, making it easier to navigate through the code and understand the project’s architecture. In this blog, we’ll explore the best practices for setting up an ideal folder and directory structure for a Django project, complete with real-world examples.
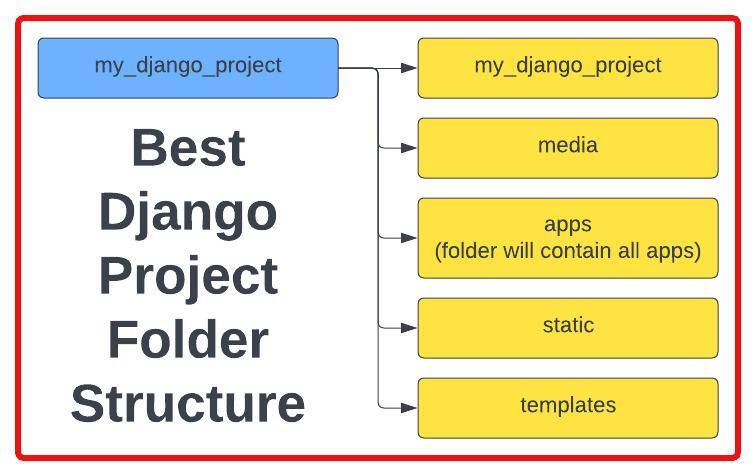
Django Folder/Directory Structuring
Note: This folder structure can also be used for structuring your Django Rest Framework project too.
1. Project Root Directory:
At the root level of your Django project, you’ll find several essential files and directories:
/my_django_project/
|-- manage.py
|-- my_django_project/
|-- settings.py
|-- urls.py
|-- asgi.py
|-- wsgi.py
|-- requirements.txt
|-- apps/
|-- static/
|-- media/
|-- templates/
manage.py
: This is a command-line utility to interact with the Django project.my_django_project/
: The inner directory with the same name as the project will hold the main settings and configurations.requirements.txt
: It contains a list of project dependencies that can be installed usingpip
.
2. The “apps” Directory:
Django projects are often composed of multiple apps, each serving a specific functionality. Grouping these apps within a dedicated “apps” directory keeps the project well-organized:
/my_django_project/
|-- apps/
|-- blog/
|-- user_management/
|-- ecommerce/
3. App Directory Structure:
Each app within the “apps” directory follows a structured layout. Here’s an example for the “blog” app:
/blog/
|-- migrations/
|-- static/
|-- templates/
|-- admin.py
|-- apps.py
|-- models.py
|-- views.py
|-- urls.py
|-- tests/
|-- forms.py (Optional)
migrations/
: Contains database migration files generated by Django for model changes.static/
: Holds static files like CSS, JavaScript, and images specific to the app.templates/
: Contains HTML templates associated with this app.admin.py
: Configures the Django admin interface for managing app models.apps.py
: App configuration and metadata.models.py
: Defines the app’s data models.views.py
: Contains the views or controller functions for handling HTTP requests.urls.py
: Defines the app’s URL patterns.tests/
: Houses unit tests for the app (optional but highly recommended).forms.py
(Optional): Includes form classes if the app requires custom forms.
4. The “static” Directory:
The global “static” directory holds project-wide static files, like CSS, JavaScript, and images used across different apps:
/my_django_project/
|-- static/
|-- css/
|-- js/
|-- images/
5. The “media” Directory:
The “media” directory is used to store user-uploaded files:
/my_django_project/
|-- media/
|-- user_uploads/
6. The “templates” Directory:
The “templates” directory stores project-wide templates and base templates that are extended by app-specific templates:
/my_django_project/
|-- templates/
|-- base.html
|-- header.html
|-- footer.html
|-- blog/
|-- user_management/
|-- ecommerce/
Common Patterns:
In addition to the above structure, some common patterns and practices include:
- Creating a separate “utils” directory for utility fuunctions and helper modules.
- Using an “api” directory for housing REST API-related files if your project includes APIs.
- Using version control, such as Git, to track changes and collaborate with a team.
Organizing your Django project with a well-thought-out folder and directory structure is essential for long-term maintainability and codebase clarity. By following the best practices and adopting the examples mentioned above, you can ensure a clean and scalable Django project that remains easy to understand and expand as your application grows.