In the domain of web development, creating user-friendly URLs that are descriptive and SEO-optimized holds immense importance. Django, a robust web framework, offers a native functionality termed “slugs,” leveraging Django’s slugify
, SlugField
, and slug
to attain this objective seamlessly. In this blog post, we will learn how to create Slug Urls in your Django Project.
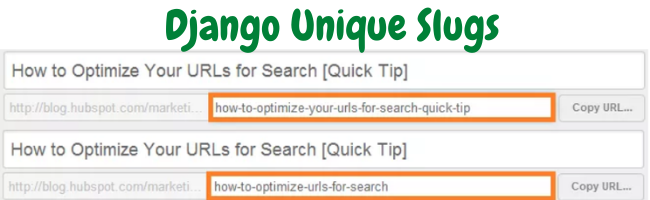
What is a Slugs in Django?
A slug is a short label or identifier used in URLs to represent a specific resource or object. It’s typically generated from the object’s title or name by removing special characters, spaces, and converting it to lowercase.
Why Are Slugs Important?
Slugs play a significant role in improving the usability and SEO optimization of your website. They make URLs more readable, descriptive, and memorable, which benefits both users and search engines.
Ways to Create Slug URL in Django:
- Using Django’s
slugify()
Function: Django provides a convenient function calledslugify()
which converts a string into a slug by replacing spaces and special characters with hyphens and converting it to lowercase. - Django-Slugify Library: The
django-slugify
library offers advanced slug generation options, allowing you to customize the behavior of slugs based on your project’s needs.
Steps to Create Slugs in Django Project using Slugify:
1. Create Slug Field: In your models, you can use the SlugField
to automatically generate slugs for objects. For example:
from django.db import models
from django.utils.text import slugify
class Article(models.Model):
title = models.CharField(max_length=100)
slug = models.SlugField(unique=True)
def save(self, *args, **kwargs):
if not self.slug:
self.slug = slugify(self.title)
super().save(*args, **kwargs)
2. Create Detail View for Slug: Here’s an example of the ArticleDetailView
implementation in Django that will create slugs automatically:
from django.shortcuts import get_object_or_404
from django.views.generic import DetailView
from .models import Article
class ArticleDetailView(DetailView):
model = Article
template_name = 'article_detail.html'
context_object_name = 'article'
slug_field = 'slug'
slug_url_kwarg = 'slug'
def get_object(self, queryset=None):
slug = self.kwargs.get(self.slug_url_kwarg)
queryset = queryset or self.get_queryset()
return get_object_or_404(queryset, **{self.slug_field: slug})
3. Utilizing Slugs in URLs: In your URL configurations, you can incorporate slugs to create user-friendly URLs:
from django.urls import path
from .views import ArticleDetailView
urlpatterns = [
path('articles/<slug:slug>/', ArticleDetailView.as_view(), name='article-detail'),
]
Best Practices and Considerations:
- Unique Slugs: Ensure that each slug is unique within its context to avoid conflicts and ensure accurate routing.
- Handling Changes: Implement a mechanism to handle changes in titles or names that could affect slugs, such as updating slugs when titles are modified.
- SEO Considerations: Craft meaningful and relevant slugs that contribute to your website’s search engine optimization efforts.
Conclusion
Django slugs are a great tool for improving both user experience and SEO on your website. By using them well, you can create clean, easy-to-read URLs that not only make navigating your site simpler for users but also boost your site’s search engine ranking.