Learn to deploy django on apache with virtualenv and mod_wsgi. Django is a Python-based web framework, that can help you to create your web application rapidly. Django is secure, powerful, fast and comes with most of the python libraries. Django is a popular choice for creating web applications. There are lots of websites that rely on Django Framework like Instagram, Mozilla, Bitbucket, Udemy, etc.
In this guide, we will help you to set up, install and deploy your Django web application on Apache Webserver. We will install and configure Django in Python Virtual Environment. We will also use Apache Virtual Host and mod_wsgi to communicate with Django over the Web Server Gateway Interface.
Python comes already installed by default on Ubuntu 14.04, 16.04 and 18.04. Now let’s see step by step guide to deploy a Django project on Apache2 Server.
Installing Required Libraries for Running Django App
We are going to download and install packages from Ubuntu Repositories which are required for Django.
We are assuming that you are using Django with Python3. Following are the commands-
sudo apt-get update sudo apt-get install python3-pip apache2 libapache2-mod-wsgi-py3
The above command will install Apache2 the Web Server, mod_wsgi for communicating and interfacing with our Django app and pip3, the python package manager for downloading python related tools.
Note – Make sure you have installed above library successfully otherwise you will get invalid command ‘wsgidaemonprocess‘ error in terminal. It will fix – invalid command ‘wsgidaemonprocess‘, perhaps misspelled or defined by a module not included in the server configuration
Configure a Python Virtual Environment
Now it is time to create Virtual Environment. The reason behind creating Virtual Environment is that it separated system tools and remove the conflicts between system packages which might occur.
We will be using virtualenv
for creating a python virtual environment. For installing virtualenv we are going to use pip3
which we have installed using the above command.
sudo pip3 install virtualenv
Creating and Configuring a Django Project
Once virtualenv
is installed, now we can structurize our project. Create a folder where ever you want and move to that folder using the terminal. We are creating a folder django_project
in the home directory.
mkdir django_project cd django_project
While inside the django_project
directory, create your virtual environment. Let’s call it env
.
virtualenv env
Now, activate the virtual environment with the following command:
. env/bin/activate
You’ll grasp it’s activated once the prefix is modified to (env)
, which can look just like the following depending on what directory you’re in:
(env) huzaif@studygyaan:~/django_project$
Within the env, install the Django package using pip3. Installing Django allows us to create and run Django applications.
pip3 install django
Now we need to Create Django Project in django_project
directory. Since we have got a project directory, we’ll tell Django to put in the files here. The key to the present is that the dot at the top that tells Django to make the files within the current directory:
django-admin startproject my_django_project .
Adjust the Project Setting and Configurations
The first factor we should always do with our fresh created project files is to change the settings.py file. Open the settings.py file which is inside the my_django_project
directory with your text editor:
nano my_django_project/settings.py
We are going to migrate all the initial data to the database. For simplicity, we will be using an SQLite database which comes default with Django. We will configure a static file directory so that it is easily served on the web.
At the bottom of the settings.py
file, we are going to add the python code to configure static file directory.
STATIC_ROOT = os.path.join(BASE_DIR, "static/")
Save and close the file when you are finished.
Complete Initial Project Setup
Using the management script which is written in the manage.py file, we will migrate our data to the Database.
cd ~/django_project python3 manage.py makemigrations python3 manage.py migrate
Create an Admin user for your project
python3 manage.py createsuperuser
For creating an admin user, you need to choose a username, email, and password of your choice.
We can gather all of the static content into the directory location we configured by commanding:
python3 manage.py collectstatic
This will collect all the project static files in static directory. It will give some output like this:
119 static files copied to '/home/huzaif/django_project/static'.
Now let’s confirm everything is working by running Django’s local webserver.
python3 manage.py runserver
If you visit http://127.0.0.1:8000/ or http://localhost:8000/ you should see the following image:
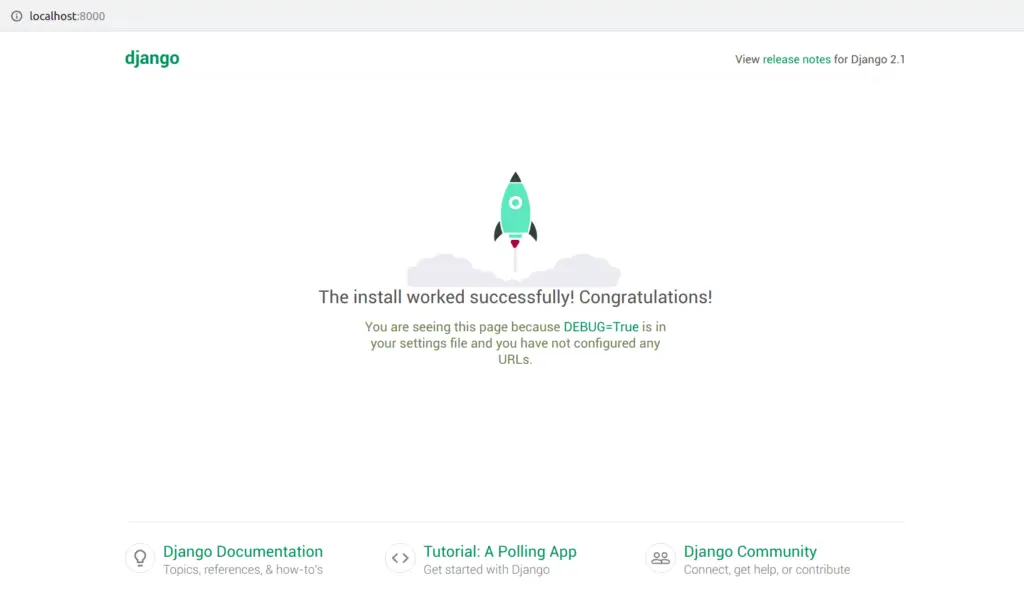
If you append admin to your URL like this http://localhost:8000/admin/, you will get a login screen where you can login with the details which you have created above.
Now we know that our project is working successfully. Lets Quit the server with CONTROL-C and temporarily get out of the virtual environment by typing
deactivate
Deploying Django Application on Apache Server
Now that your project is working perfectly fine, its time to deploy Django Application on the Web server. The client connections that it receives are going to be translated into the WSGI format that the Django application expects the mistreatment of the mod_wsgi module.
Let’s create a virtual host file for our project. With your text editor create a .conf
file in apache2 /etc/apache2/sites-available/
directory.
sudo nano /etc/apache2/sites-available/djangoproject.conf
Add the following text to djangoproject.conf
file:
<VirtualHost *:80>
ServerAdmin [email protected]
ServerName djangoproject.localhost
ServerAlias www.djangoproject.localhost
DocumentRoot /home/user/django_project
ErrorLog ${APACHE_LOG_DIR}/error.log
CustomLog ${APACHE_LOG_DIR}/access.log combined
Alias /static /home/user/django_project/static
<Directory /home/user/django_project/static>
Require all granted
</Directory>
Alias /static /home/user/django_project/media
<Directory /home/user/django_project/media>
Require all granted
</Directory>
<Directory /home/user/django_project/my_django_project>
<Files wsgi.py>
Require all granted
</Files>
</Directory>
WSGIDaemonProcess django_project python-path=/home/user/django_project python-home=/home/user/django_project/env
WSGIProcessGroup django_project
WSGIScriptAlias / /home/user/django_project/my_django_project/wsgi.py
</VirtualHost>
When you are finished making the bellow changes, save and close the file.
Note: You need to change in the above code user to your username. To find your username use this command
whoami
We are having a project directory like this:
django_project └── env (All ENV File) ├── manage.py └── my_django_project ├── init.py ├── settings.py ├── urls.py └── wsgi.py
Where django_project
is the main directory and my_django_project
is subdirectory in it. Change the directories in the above code respectively.
One example I will show you.
/home/user/django_project/my_django_project/wsgi.py
- change
user
to yourusername
django_project
with your main directory namemy_django_project
with your subdirectory directory name
Somewhat like this:
/home/username/main_django_directory/django_subdirectory/wsgi.py
In the above .conf
the file we are keeping project URL as djangoproject.localhost
. If you want to change then change it.
Tip: If you are getting this error – Invalid command ‘WSGIDaemonProcess’, perhaps misspelled or defined by a module not included in the server configuration
Then the explanation for obtaining the error may well be that you simply haven’t run this command
sudo apt-get install python3-pip apache2 libapache2-mod-wsgi-py3
Enable the Virtual Host File for Django Project
Once we created djangoproject.conf
the file we need the enable that virtual host file by typing
cd /etc/apache2/sites-available sudo a2ensite djangoproject.conf
The above command will give output something like this –
Enabling site djangoproject. To activate the new configuration, you need to run: service apache2 reload
Now we will Set Up Local Hosts File. This is optional but recommended.
sudo nano /etc/hosts
And add the below line at the end of file and save it.
127.0.0.1 djangoproject.localhost
Note: We will be to access our Django project using this URL http://djangoproject.localhost/
Wrapping Up Some Permissions Issues
sudo ufw allow 'Apache Full'
If you are using SQLite as your database then we need to give some more permissions
sudo chmod 664 /home/user/django_project/db.sqlite3 sudo chown :www-data /home/user/django_project/db.sqlite3
sudo chown :www-data /home/user/django_project
Check your Apache files to make sure you did not make any syntax errors:
sudo apache2ctl configtest
It will output – Syntax OK
which means no error and everything is working.
Back to our Project Setup
Go back to the directory where you have kept your Django project. We have kept our project in home
, django_project
directory
cd django_project/
Activate the Virtual Environment which we have created previously
source env/bin/activate
Edit your setting.py
file again to add allowed host URL that we have created using the virtual host file
sudo nano my_django_project/settings.py
Add the URL in ALLOWED_HOST
List which is above INSTALLED_APPS
.
ALLOWED_HOSTS = ['djangoproject.localhost']
Save and Exit the file.
Now we need to run the run server by typing runserver
command
python3 manage.py runserver
Quit the server with CONTROL-C. This will not stop the server from running. When you get out, you need to restart Apache to make these changes take effect:
sudo service apache2 restart
Hurray, you are done. That’s it now you just need to go to the URL of your project. In our case http://djangoproject.localhost/.
You will see the just like below image.
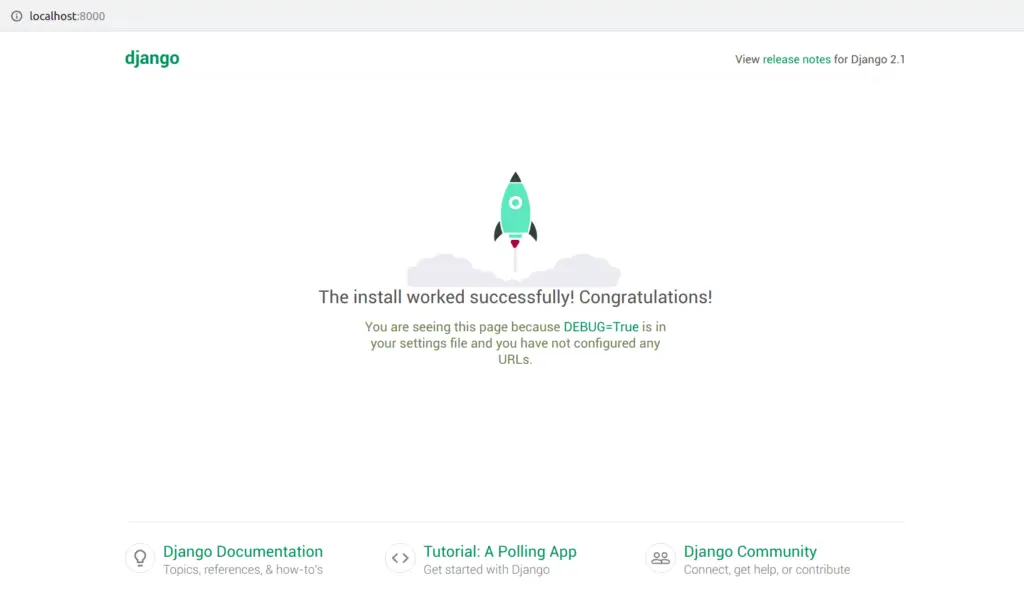
Tips for Running Django Project on Apache Server
Tip 1. When I was running the project for the first time I was facing some issues like changes in code were not reflecting on the browser. To make changes reflect, you need to restart your apache server.
sudo service apache2 restart
Tip 2. Once your Django Application is running, then you might not need to use runserver
command. Django Application runs forever and if some issues then you need to rectify your code and restart your apache server.
Tip 3. If you are getting read-write operation problems, then you might give read-write permissions.
Tip 4. Most Important Tip. If you want to see the error of Django Application, you can get those error at Apache Error Log. See the Django errors in terminal using the below command:
tail -f /var/log/apache2/error.log