Learn to use socketio with django. This tutorial is update to django-socketio. In this tutorial i will explain how to use python-socketio Library with Django Integrations. Learn how to integrate Python Socket IO with Django WsGi Server and build real time applications like games, chat, chatbot. This is an alternative to Django Channels where it uses websockets.
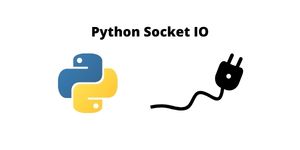
Intergrating Python Socket IO with Django WSGI Application
Install SocketIO Dependencies
Create a Django project and Add following in the .txt
and install all the dependencies.
dnspython==1.16.0 enum-compat==0.0.3 eventlet==0.25.2 greenlet==0.4.16 monotonic==1.5 python-engineio python-socketio pytz==2020.1 six==1.15.0
Example – pip install -r requirements.txt
Django with SocketIO
Create a socket app in Django project and add it to INSTALLED_APPS
.
INSTALLED_APPS = [ 'socketio_app', .... ]
Now inside socketio_app
, edit views.py
and add following code. Download views.py file from here. This code will create socket events for your applications.
In your urls.py
file add following path
from socketio_app.views import index urlpatterns = [ path('', views.index, name='index'), ]
In your socketio_app
folder, create a static
folder and inside that add the following index.html
file. Download index.html file from here.
Edit WSGI File for Python SocketIO
Note that socketio runs in async mode i.e. it will not be part of the request response process. Hence, we have mentioned async_mode='eventlet'.
Eventlet is the module which will spawn a new thread for each connection. We will talk more about it in the deployment section.
Apart from this, we need to override callback methods if we want to do something when connection is established or disconnected.
Add following code in wsgi
file for connecting to the client
from socketio_app.views import sio import socketio application = socketio.WSGIApp(sio, application) import eventlet import eventlet.wsgi eventlet.wsgi.server(eventlet.listen(('', 8000)), application)
Note – While running server, if eventlet ask permission, then allow it.
Now you can run the server.
Static Files Not Serving Using Evenlet and Socket IO
If you project is not able to serve static file using eventlet server. Do following changes in your wsgi.py file.
import os from django.core.wsgi import get_wsgi_application import socketio from django.contrib.staticfiles.handlers import StaticFilesHandler from apps.chatbox.views import sio os.environ.setdefault("DJANGO_SETTINGS_MODULE", "chatbot.settings") django_app = StaticFilesHandler(get_wsgi_application()) application = socketio.Middleware(sio, wsgi_app=django_app, socketio_path='socket.io') import eventlet import eventlet.wsgi eventlet.wsgi.server(eventlet.listen(('', 8000)), application)
Find this project on GitHub.