QR (Quick Response) codes have become ubiquitous in today’s digital landscape. They provide an efficient way to store and share information, such as URLs, contact details, or other data. In this tutorial, we’ll explore how to generate QR codes in a Django web application. We’ll walk through the process, step by step, and provide examples to help you implement QR code generation in your Django project.
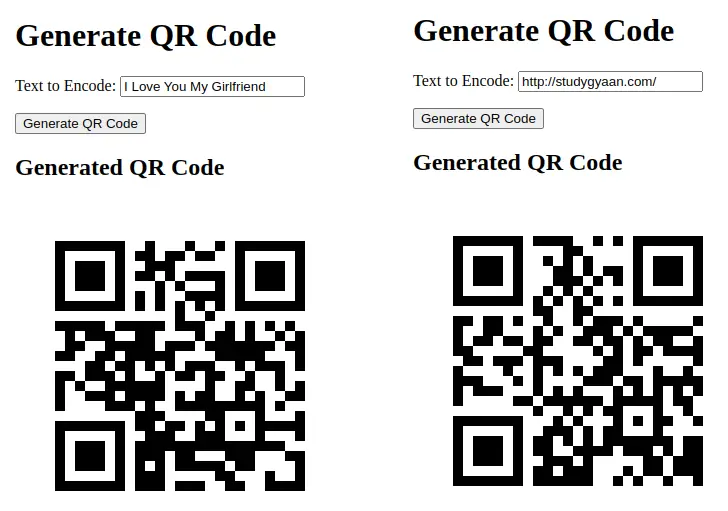
Prerequisites
Before we begin, make sure you have the following prerequisites:
- Python and Django installed on your system.
- The
qrcode
library for generating QR codes. You can install it using pip:
pip install qrcode
Certainly, if you want to generate QR codes without using a model and display the QR code on the same HTML page, you can simplify the process. Here’s an updated example:
Generate QR Code in Python Django using Base64 Image
We will generate QR code using Base64 Image, so no need to store image. Lets get started!
Step 1: Create a Django Project and App
Start by creating a new Django project and app:
django-admin startproject qrcode_project
cd qrcode_project
python manage.py startapp qrcode_app
Step 2: Create a Django Form
In qrcode_app/forms.py
, create a form for collekting the text data you want to encode into a QR code:
# qrcode_app/forms.py
from django import forms
class QRCodeForm(forms.Form):
text_data = forms.CharField(label='Text to Encode', max_length=255)
Step 3: Create a View
In qrcode_app/views.py
, create a view that generates the QR code and displays it on the same page. It create a temporary image file and serve it as a base64-encoded string in the template
# qrcode_app/views.py
from django.shortcuts import render
import qrcode
from qrcode.image.pil import PilImage
from PIL import Image
import io
import base64
from .forms import QRCodeForm
def generate_qrcode(request):
qr_code_image = None
if request.method == 'POST':
form = QRCodeForm(request.POST)
if form.is_valid():
text_data = form.cleaned_data['text_data']
# Generate the QR code
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4,
)
qr.add_data(text_data)
qr.make(fit=True)
qr_code_image = qr.make_image(fill_color="black", back_color="white").convert('RGB')
# Create a BytesIO buffer to temporarily store the image
buffer = io.BytesIO()
qr_code_image.save(buffer, format="PNG")
qr_code_image_data = base64.b64encode(buffer.getvalue()).decode()
else:
form = QRCodeForm()
return render(request, 'generate_qrcode.html', {'form': form, 'qr_code_image_data': qr_code_image_data})
Step 4: Create a Template
Create a template in the qrcode_app/templates/
directory to display the form and the generated QR code (generate_qrcode.html
):
<!DOCTYPE html>
<html>
<head>
<title>Generate QR Code</title>
</head>
<body>
<h1>Generate QR Code</h1>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Generate QR Code</button>
</form>
{% if qr_code_image_data %}
<h2>Generated QR Code</h2>
<img src="data:image/png;base64,{{ qr_code_image_data }}" alt="QR Code">
{% endif %}
</body>
</html>
Step 5: Configure URLs
Configure the URL routing for your app by adding URL patterns in qrcode_app/urls.py
:
from django.urls import path
from . import views
urlpatterns = [
path('generate/', views.generate_qrcode, name='generate_qrcode'),
]
Include the app’s URLs in your project’s urls.py
:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('qrcode/', include('qrcode_app.urls')),
]
Step 6: Run the Development Server
Run the Django development server:
python manage.py runserver
Visit http://127.0.0.1:8000/qrcode/generate/
in your browser. You will see a form where you can enter text data. Upon submission, the page will display the generated QR code without using a model.
This approach ensures that the generated QR code image is temporarily stored in memory and served as a base64-encoded string in the template, allowing it to be displayed without saving it as a file on the server.
Conclusion
With these steps, you created a Django web application that generates QR codes and displays them on the same page.. Users can input text data, submit the form, and immediately see the generated QR code. This approach simplifies the process and can be used for various quick QR code generation needs in your Django projects
Find this project on Github.
Blogs You Might Like to Read!
- Create Django News Website using Python News Api
- How to Build Language Translator App using Python Django
- Django Project: Text to HTML and HTML to Text Generator/Converter
- Folder and File Structure for Django Templates: Best Practices
- Build a Weather App in Python Django: Guide with Example
- Best Folder and Directory Structure for a Django Project