In this tutorial, we will guide you through the process of creating a news app in Django from scratch. We’ll use the newsapi-python
library to fetch news articles from an external source. By the end of this tutorial, you will have a basic news application that can display news articles on a web page.
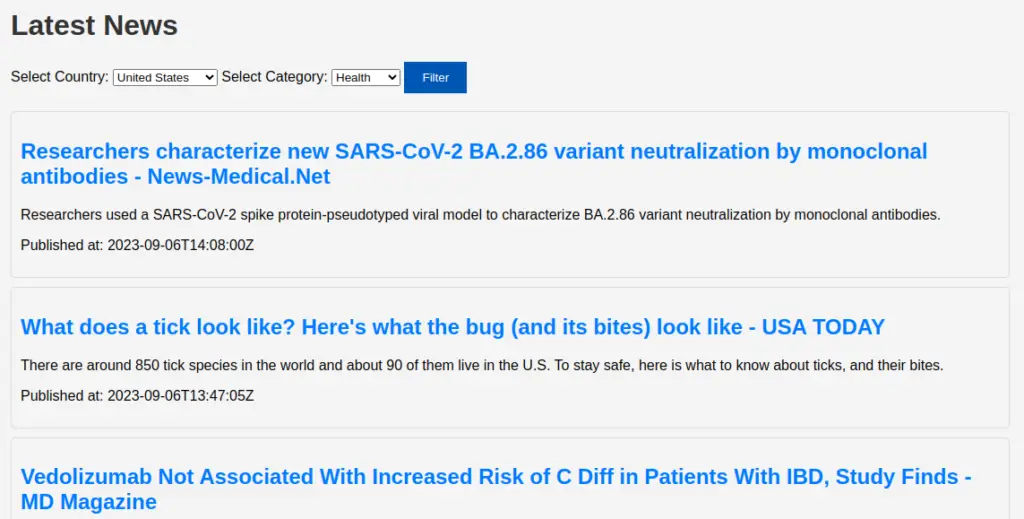
Prerequisites
Before we begin, make sure you have the following prerequisites:
- Python and Django installed on your system
- An API key from the News API (you can get a free key by signing up). Click on Get API Key and Sign Up.
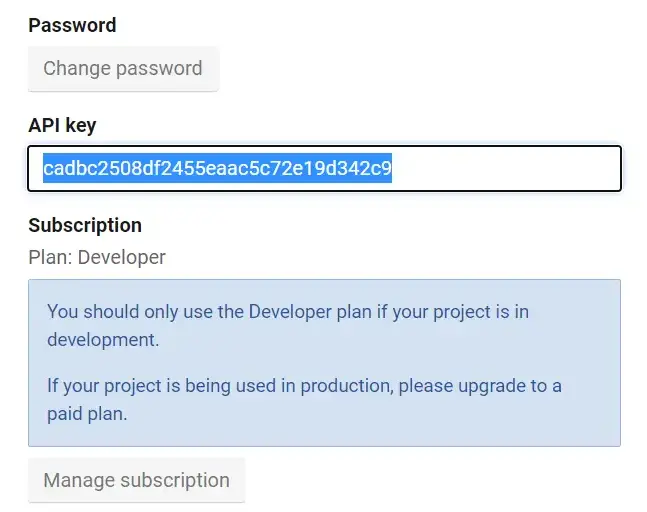
Implementation
Step 1: Create a Django Project and App
Let’s start by creating a new Django project and app:
django-admin startproject newsapp_project
cd newsapp_project
python manage.py startapp newsapp
To fetch news data from the News API using the newsapi-python
library. You’ll need to install this library using pip
:
pip install newsapi-python
Step 2: Configure Django Settings
Open the settings.py
file in your project directory and add 'newsapp'
to the INSTALLED_APPS
list:
pythonCopy code
INSTALLED_APPS = [
# ...
'newsapp',
]
Step 3: Create a Django Form
First, create a Django form to collect the user’s selection for country and category. In the newsapp/forms.py
file, define your form:
# newsapp/forms.py
from django import forms
class NewsFilterForm(forms.Form):
COUNTRIES = [
('us', 'United States'),
('gb', 'United Kingdom'),
('ca', 'Canada'),
# Add more countries as needed
]
CATEGORIES = [
('general', 'General'),
('business', 'Business'),
('health', 'Health'),
# Add more categories as needed
]
country = forms.ChoiceField(choices=COUNTRIES, label='Select Country')
category = forms.ChoiceField(choices=CATEGORIES, label='Select Category')
Step 4: Create Django Views to Fetch Data from API
Now, let’s create views and templates to display the news artaicles on a web page. Define a view in newsapp/views.py
:
from django.shortcuts import render
from newsapi import NewsApiClient
from .forms import NewsFilterForm
def news_list(request):
api_key = 'YOUR_NEWS_API_KEY' # Replace with your News API key
newsapi = NewsApiClient(api_key=api_key)
# Default values for country and category
country = 'us'
category = 'general'
if request.method == 'GET':
form = NewsFilterForm(request.GET)
if form.is_valid():
country = form.cleaned_data['country']
category = form.cleaned_data['category']
# Fetch news articles based on user selections
articles = newsapi.get_top_headlines(country=country, category=category, page_size=10)
return render(request, 'news_list.html', {'articles': articles['articles'], 'form': form})
Step 5: Create the Template
Create your template file newsapp/templates/news_list.html
to include the form for selecting country and category:
<!DOCTYPE html>
<html>
<head>
<title>News App</title>
</head>
<body>
<h1>Latest News</h1>
<form method="get">
{{ form.as_p }}
<button type="submit">Filter</button>
</form>
<ul>
{% for article in articles %}
<li>
<h2><a href="{{ article.url }}">{{ article.title }}</a></h2>
<p>{{ article.description }}</p>
<p>Published at: {{ article.publishedAt }}</p>
</li>
{% endfor %}
</ul>
</body>
</html>
Here is html file with styling.
Step 6: Configure URLs
Keep the URL routing configuration in newsapp/urls.py
as it is:
from django.urls import path
from . import views
urlpatterns = [
path('', views.news_list, name='news_list'),
]
Include the app’s URLs in your project’s urls.py
:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('newsapp.urls')),
]
Step 7: Run the Development Server
Run the Django development server:
python manage.py runserver
Visit http://127.0.0.1:8000/
in your browser, and you should see a list of the latest news articles fetched from the News API and displayed directly on the web page.
Conclusion
In this tutorial, you’ve learned how to create a basic news app in Django from scratch. You’ve set up a Django project, fetched news data from an external API, stored it in a database, and displayed it on a web page. From here, you can further enhance your news app by adding features like search, category filters, and user authentication This project serves as a solid foundation for building a more comprehensive news application
Find this project on Github.
Blogs You Might Like to Read!
- How to Build Language Translator App using Python Django
- Django Project: Text to HTML and HTML to Text Generator/Converter
- How to Generate QR Code in Python Django
- Folder and File Structure for Django Templates: Best Practices
- Django Bootstrap – Integrate Template Example
- Best Folder and Directory Structure for a Django Project
- Build a Weather App in Python Django: Guide with Example