Docker can read instructions from a Dockerfile and generate images for you automatically. A Dockerfile is a text file that contains all of the commands that a user may use to construct an image from the command line. Users may use docker build to automate a build that performs many command-line instructions in a row.
Docker Files are basically scripts that you can write and then build into docker image. Then image can then be run to create the Container. Its similar to shell scripts
Before writing the dockerfile, lets understand the syntax and format.
- FROM <base_image>
- ADD <source> <destination>
- COPY <source> <destination>
- RUN <command>
- WORKDIR <directory>
- CMD <command>
- VOLUME <path>
- EXPOSE <ports>
- ENTRYPOINT <command> <parameter_1> <parameter_2>
- LABEL <key>=<value>
Previous Tutorial – How to use Docker Hub and Registry
Create Dockerfile Instructions
Let us consider we are in a empty folder, inside the folder create a file with extension dockerfile.
Example – example.dockerfile
For this example, we will execute a python file in ubuntu. Create a python file with name main.py
and add bellow code in it.
# main.py print('Running using docker file...')
Put the bellow code in example.dockerfile
save it.
FROM ubuntu:latest WORKDIR /app ADD . /app RUN apt update && apt install python -y CMD python /app/main.py LABEL color=red
Lets understand the dockerfile script – We are pull the ubuntu image, then creating working directory named app
. Once the directory is create we are copying all the files which are in current directory to app
directory. Then running the ubuntu update command and installing python in it. Once apt run, we are commanding python to execute our main.py file and create a label color.
Build Docker Image using DockerFile
Once you have follow above steps. Run the bellow build
command using example.dockerfile
docker build -t custom-py -f new.dockerfile .
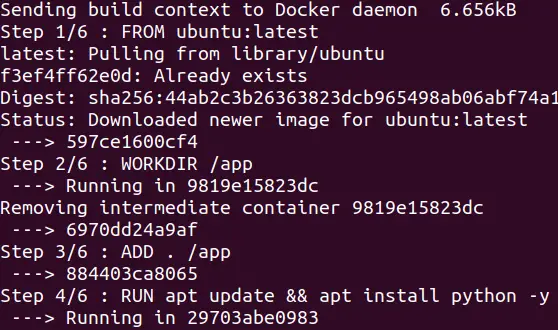
If everything goes fine, you can chech your docker image using bellow code.
docker images
Best Practices for Docker File
You can also go through official docker website for best practices.
Some points which i think are importants –
- We can use
&&
to run multiple commands - Use
.dockerignore
file to ignore unwanted things
Next Tutorial