In modern web applications, providing users with a seamless and efficient search experience is crucial. Autocomplete search with suggestions is a feature that can significantly enhance the user experience, making it easier for users to find what they’re looking for quickly. In this tutorial, we’ll explore how to implement autocomplete search with suggestions in a Django web application.
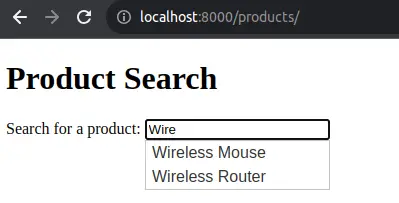
Before diving into implementation, lets understand where we can use autocomplete.:
- Search Bars: Autocomplete can be used to suggest search queries or products as users type in a search bar.
- Address Forms: It can assist users in entering addresses by suggesting city names, postal codes, or street names.
- Email Addresses: When users enter recipient email addresses, autocomplete can suggest previously used addresses from their contact list. In registration or login forms, autocomplete can suggest usernames or user IDs that match the characters typed.
- Product Selection: In e-commerce applications, it can suggest products based on user input, making it easier to find and select items.
- Tags or Categories: When tagging content or selecting categories, autocomplete helps users find relevant options quickly.
Implementing AutoComplete Search Functionality using Django and JQuery
In this example, we’ll create a Django web application named “Products” and implement autocomplete search with suggestions for product names.
Step 1: Setting up the Django Project
Create a new Django project and a Django app:
django-admin startproject myproject
cd myproject
python manage.py startapp products
Add the products
app to your project’s settings in settings.py
:
INSTALLED_APPS = [
# ...
'products',
# ...
]
Step 2: Creating a Django Model
In the products
app, create a models.py
file:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
def __str__(self):
return self.name
Run migrations to apply the changes:
python manage.py makemigrations
python manage.py migrate
Note: For demo purpose, we have add products using admin panel by creating superuser login.
Step 3: Implementing Autocomplete Search with Django
In this step, we’ll set up the Django view and URL to handle the autocomplete search. Lets create a product page and ProductAutocomplete which returns JSON response. In your products
app, add following code in views.py
from django.http import JsonResponse
from django.views import View
from .models import Product
from django.shortcuts import render
def product_search(request):
return render(request, 'index.html')
class ProductAutocomplete(View):
def get(self, request):
query = request.GET.get('term', '')
products = Product.objects.filter(name__icontains=query)[:10]
results = [product.name for product in products]
return JsonResponse(results, safe=False)
Define a URL pattern for view in the urls.py
of the products
app: Create urls.py in your app
from django.urls import path
from . import views
urlpatterns = [
path('', views.product_search, name='product-search'),
path('autocomplete/', views.ProductAutocomplete.as_view(), name='product-autocomplete'),
]
Add the path of product urls in main urls.py
which resides in myproject/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('products/', include('products.urls')),
]
Step 4: Adding Suggestions to the Autocomplete Search and Enhancing Interface
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
We will include above jQuery and jQuery UI libraries in your project’s template. In your Django app’s template (e.g., products/templates/index.html
), add the following code:
<!DOCTYPE html>
<html>
<head>
<title>Product Search</title>
<!-- Include jQuery and jQuery UI -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body>
<h1>Product Search</h1>
<form>
<label for="search">Search for a product:</label>
<input type="text" id="search" name="search">
</form>
<script>
$(function() {
$("#search").autocomplete({
source: "{% url 'product-autocomplete' %}",
minLength: 2, // Minimum characters before triggering autocomplete
});
});
</script>
</body>
</html>
Step 6: Testing the Autocomplete Search
Start the Django development server:
python manage.py runserver
Visit http://localhost:8000/products/
in your web browser. You should see the product search input field. As you type, the autocomplete suggestions should appear below the input.
Conclusion
1Congratulations! You’ve implemented autocomplete search with suggestions for product names in your Django web application. You can customize this example further to fit your specific use case, such as styling the suggestions or connecting them to actual product details.
Find this project on Github.