Django REST Framework is a robust and flexible toolkit for building Web APIs. It is written in python and allows the user to create some cool and awesome web applications. Most of the developers are very good in technical stuff, but are not so good in writing, and as we know documentation plays a very important role in any development. So if you are using Django and want to write documentation for the API then you don’t have to worry because django will create it for you. In this blog we will learn how to create API Documentation in Django REST Framework. For creating documentation we will use a python module drf-yasg. You can refer to this documentation to understand drf-yasg.
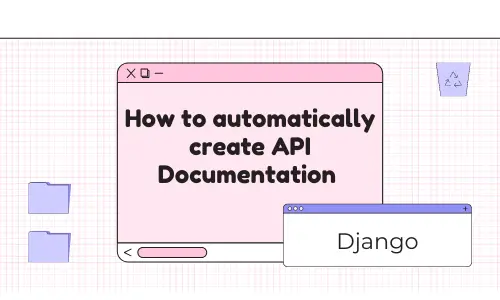
Implementations
Step 1. Create Django Project
We are first going to create a django project and an app inside that project .
1. Create a Django project.
django-admin startproject blog23
2. Create an app in that django-project.
python manage.py startapp documentation
3. Install drf-yasg by running the following command in your terminal.
pip install drf-yasg
4. Add your app name and drf-yasg in installed apps.
Settings.py
INSTALLED_APPS = [ 'documentation', ‘drf-yasg’, 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', ]
Step 2. Add files and Folder to the Django Project
We need to add a urls.py file in the app folder and have to add some configuration in django_project (here,blog23) > urls.py.
- Create a new file in the app folder(here, documentation) save it with the name urls.py .
- Add path for this url.py file in blog23 > urls.py .
Urls.py
from django.contrib import admin from django.urls import path,include urlpatterns = [ path('admin/', admin.site.urls), path('',include('documentation.urls')) ]
3. Now we need to add driver code for drf-yasg in blog23 > urls.py .
Urls.py
from rest_framework import permissions from drf_yasg.views import get_schema_view from drf_yasg import openapi from django.contrib import admin from django.urls import path,include from django.conf.urls import url schema_view = get_schema_view( openapi.Info( title="Test API", default_version='v1', description="Test description", terms_of_service="https://www.google.com/policies/terms/", contact=openapi.Contact(email="[email protected]"), license=openapi.License(name="BSD License"), ), public=True, permission_classes=(permissions.AllowAny,), ) urlpatterns = [ url(r'^playground/$', schema_view.with_ui('swagger', cache_timeout=0), name='schema-swagger-ui'), url(r'^docs/$', schema_view.with_ui('redoc', cache_timeout=0), name='schema-redoc'), path('admin/', admin.site.urls), path('',include('documentation.urls')) ]
Step3. API documentation
- Run migrations with the help of the following command.
python manage.py makemigrations
python manage.py migrate
2. Create a function for reading the doc in app_name ( here, documentation) > views.py.
Views.py
from django.shortcuts import render from drf_yasg.utils import swagger_auto_schema from rest_framework import status, permissions, serializers from rest_framework.response import Response from rest_framework.views import APIView # simple serializer for student class StudentForm(serializers.Serializer): name = serializers.CharField() address = serializers.CharField() # simple endpoint to take the serializer data class Student(APIView): permission_classes = (permissions.AllowAny,) @swagger_auto_schema(request_body=StudentForm) def post(self, request): serializer = StudentForm(data=request.data) if serializer.is_valid(): json = serializer.data return Response( data={"status": "OK", "message": json}, status=status.HTTP_201_CREATED, ) return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
3. Provide a path for the above function in app_name ( here, documentation ) > urls.py.
Urls.py
from django.urls import path from . import views urlpatterns = [ path('',views.Student.as_view(),name="sendemail") ]
When you run http://127.0.0.1:8000/docs/ you can view the api documentation.
Output :-
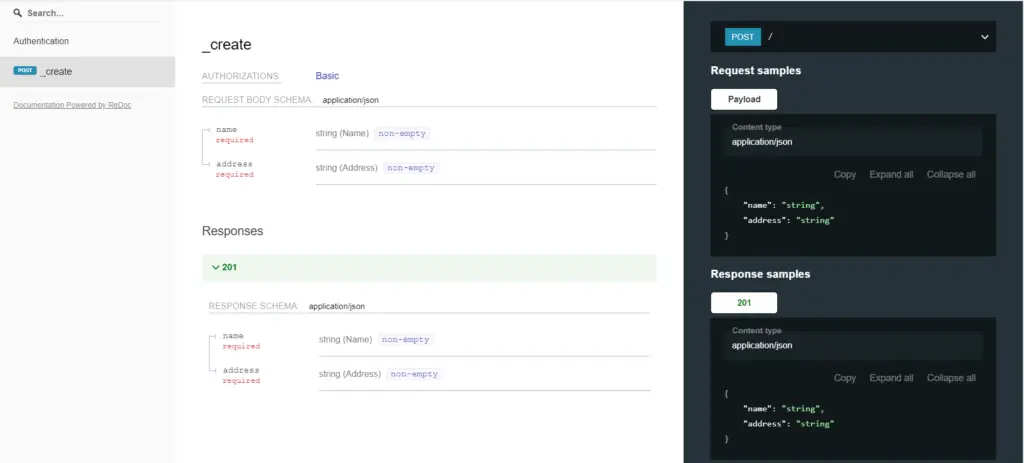
When you run http://127.0.0.1:8000/playground/ you can view the playground section.
Output :-
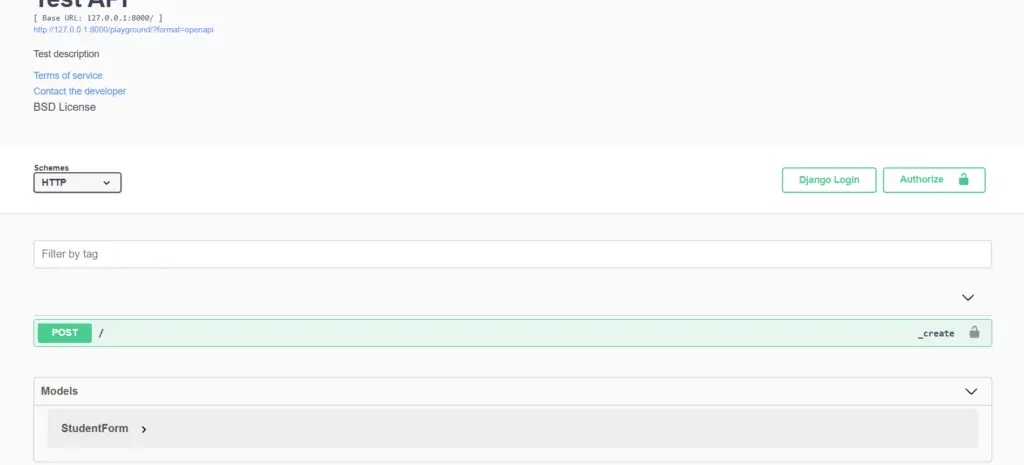
Quick Revision :-
- Create your django project folder.
- Create an app folder in the django project folder.
- Install drf-yasg.
- Add app name and drf-yasg in the installed_app section of django_folder > settings.py .
- Add file named as urls.py in the app folder and provide its path in django_project > urls.py.
- Run migrations.
- Create a function for reading documents in app_folder > views.py.
- Add path of index html page in app_folder > urls.py.
GitHub Link :-
https://github.com/jaya2320/blog_api_documentation
Conclusion
So, we learned how to create API Documentation in Django. I hope this blog will be useful for you and helps you to understand each and every step. Hope all your doubts get cleared. Thank you