Django REST Framework is a robust and flexible toolkit for building Web APIs. It is written in python and allows the user to create some cool and awesome web applications. In this blog we will learn about integrating facebook like and share plugin using Django. If you have ever used facebook you know that below every post there is a like and share button. You might come across some of your projects where you would like to integrate them, so this is the right place for you let’s get started.
Implementations
Step 1. Create Django Project
We are first going to create a django project and an app inside that project .
- Create a Django project.
django-admin startproject blog21
2. Create an app in that django-project.
python manage.py startapp fblikebutton
3. Add your app name in installed apps.
Settings.py
INSTALLED_APPS = [
'fblikebutton',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
]
Step 2. Add files and Folder to the Django Project
We need to create a template folder in the django folder and a urls.py file in the app folder.
- Create a new folder in the django folder(here,blog21) save it with the name template.
- Add the path for this template folder in blog21 > settings.py .
Settings.py
import os
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [os.path.join(BASE_DIR,'template')],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
3. Create a new file in the app folder(here, fblikebutton) save it with the name urls.py .
4. Add path for this url.py file in blog21 > urls.py .
Urls.py
from django.contrib import admin
from django.urls import path,include
urlpatterns = [
path('admin/', admin.site.urls),
path('',include('fblikebutton.urls'))
]
Step3. Integrate Fb Like and Share button
- Create an html file.
Index.html
<html>
<head>
<title>
FB Like and Share button
</title>
</head>
<body>
<h1>Please hit the like and Share Button</h1>
</body>
</html>
2. Go to the link given below and click on the button “Get Code”.
https://developers.facebook.com/docs/plugins/like-button/
3. Copy the code and follow the instructions i.e. you have to add the first code inside your body tag and the second code wherever you want your plugins to appear. Below is the picture of how the code will look like on the link given in the above step.
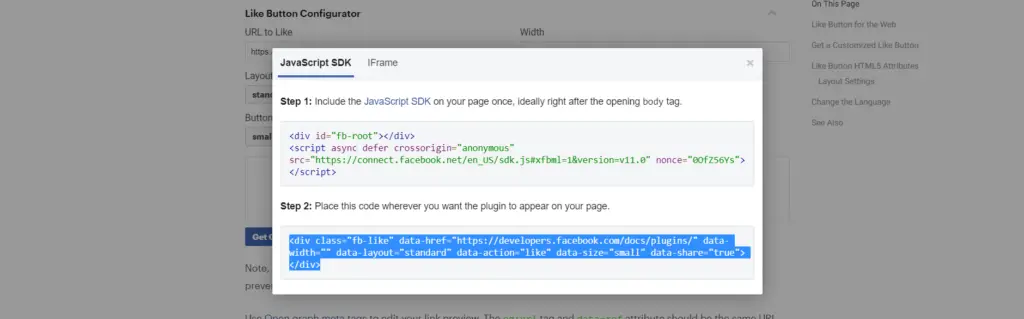
The updated html file will appears to be
Index.html
<html>
<head>
<title>
FB Like and Share button
</title>
</head>
<body>
<h1>Please hit the like and Share Button</h1>
<div id="fb-root"></div>
<script async defer crossorigin="anonymous" src="https://connect.facebook.net/en_US/sdk.js#xfbml=1&version=v11.0"
nonce="0OfZ56Ys"></script>
<div class="fb-like" data-href="https://developers.facebook.com/docs/plugins/" data-width="" data-layout="standard"
data-action="like" data-size="small" data-share="true"></div>
</body>
</html>
4. Provide a path for the above html file in app_name ( here, fblikebutton) > urls.py.
Urls.py
from django.urls import path
from . import views
urlpatterns = [
path('',views.index,name="index"),
]
5. Create function for the path provided in urls file in app_name ( here, fblikebutton) > views.py.
Views.py
from django.shortcuts import render
# Create your views here.
def index(request):
return render(request,'index.html')
Output :-
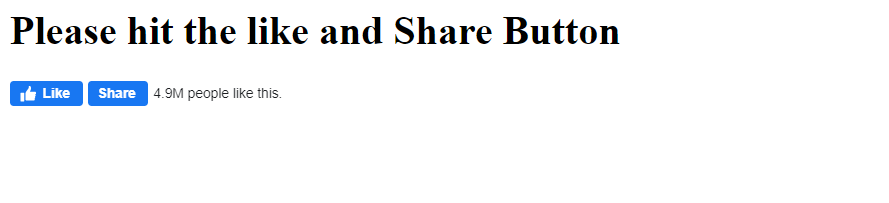
Quick Revision :-
- Create your django project folder.
- Create an app folder in the django project folder.
- Add template folder in the django folder and provide its path in django_folder > settings.py .
- Create file named as urls.py in the app folder and provide its path in django_project > urls.py.
- Add html code for the in django_project > template.
- Add path of index html page in app_folder > urls.py.
- Create function for the index html page in app_folder >views.py.
GitHub Link :-
https://github.com/jaya2320/blog_fb_like_and_share_button
Conclusion :-
So, we learned about integrating facebook like and share plugin using Django. I hope this blog will be useful for you and helps you to understand each and every step. Hope all your doubts get cleared. Thank you.